HTML Basics
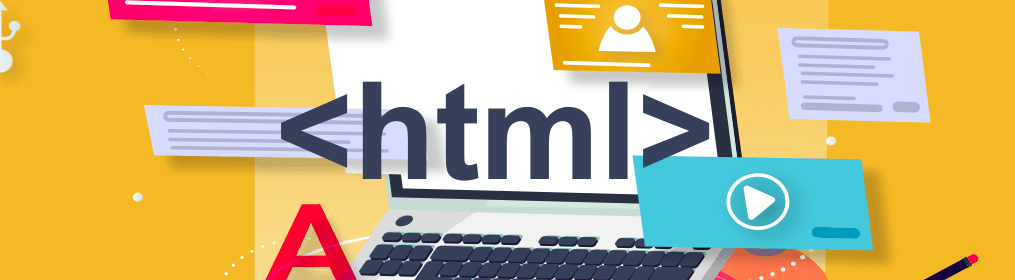
Navigation system works on the concept of linked list.
1. Introduction:
What is HTML?
- HTML, which stands for Hypertext Markup Language. It is the standard markup language used in creating web pages. It is the backbone of any website and is used to format the content on the web page.
- Web pages created with HTML can include text, images, links, forms, audio, video and more.
- HTML is not a programming language, meaning it does not have logic like if…else statements or loops. It is a markup language and is used to structure content on the web.
What is a website?
A website is essentially a folder which contains HTML, CSS and javascript documents as well as images, videos, audios, texts and more…
Basic HTML structure
|
|
Basic HTML syntax
- standard HTML Elements
- Self closing HTML Elements
|
|
2. HTML elements
Heading and paragraphs
HTML provides six levels of headings, from <h1>
to <h6>
. These tags are used to create headings of different sizes and provide a visual hierarchy to your content.
The <p>
tag is used to define paragraphs of text. It separates blocks of text and helps maintain readability and organization.
|
|
This is a h1 heading
This is a h2 heading
This is a h3 heading
This is a h4 heading
This is a h5 heading
This is a h6 heading
This is a paragraph of text.
Lists
HTML offers two types of lists: ordered lists <ol>
and unordered lists <ul>
. Ordered lists are used for items with a specific order or sequence, while unordered lists are used for items without a particular order. Each list item is defined using the <li>
tag.
|
|
- Item 1
- Item 2
- Item 3
Tables
Tables <table>
are used to organize tabular data. They consist of rows <tr>
and cells <td>
. Tables help present data in a structured format.
|
|
Cell 1 | Cell 2 |
Cell 3 | Cell 4 |
Divs
The <div>
element is a versatile container that allows you to group and style sections of your webpage. It provides flexibility in organizing and styling content. It is primarily used for creating sections and grouping elements.
|
|
Section Title
This is another paragraph inside a div.
Span
The <span>
element is a versatile inline container that allows you to group and style specific sections of text or inline elements. It is primarily used for targeting and styling specific sections of text or inline elements within your HTML content.
|
|
Comments in HTML
Comments in HTML allow you to leave notes in yout code that are ignored by the browser.
|
|
this paragraph contains some text.
Block and inline elements
Block-Level Elements:
- Block-level elements start on a new line and occupy the full width available by default.
- Examples of block-level elements include
<div>, <p>, <h1> to <h6>, <ul>, <li>, and <section>
. - Block-level elements create a visual block or box-like structure.
- Block-level elements can contain other block-level and inline-level elements.
- They are commonly used for structuring and grouping content, creating sections, and adding vertical spacing.
Inline-Level Elements:
- Inline-level elements do not start on a new line and only occupy the necessary width to render their content.
- Examples of inline-level elements include
<span>, <a>, <strong>, <em>, <img>, and <input>
. - Inline-level elements flow within the surrounding text and do not create line breaks.
- Inline-level elements cannot contain block-level elements but can contain other inline-level elements.
- They are commonly used for styling or adding emphasis within a line of text or to create inline links or images.
3. HTML Attributes
Attribute basics
- All HTML elements can have attribute, but some attributes can be applied to all elements and some are specific to particular elements.
- Attributes provide additional information about elements.
- Attributes are always specified in the start tag.
- Attributes usually come in name/value pairs like:
name="value"
. - Attributes values ate case sensitive, which means that
class="some-name"
is not the same asclass="some-Name"
.
Class attribute
The class attribute is used to assign a specific class to an element. It is primarily used for styling purposes.
|
|
ID attribute
The id attribute provides a unique identifier for an element on a webpage. It allows to target and manipulate specific elements using JavaScript and CSS.
|
|
Each id must be unique within the HTML document.
Src attribute
The src attribute is used with <img>
tag to specify the source URL of an image. It enables you to display images on your webpage.
|
|
Alt attribute
The alt attribute is used with the <img>
tag to provide alternative text for an image. It is displayed if the image fails to load or for accessibility purpose, enabling screen readers to describe the image to visually impaired users.
|
|
Href attribute
The href attribute is used with <a>
tag to specify the destination URL or location of a hyperlink. It allows you to create clickable links that navigate to other web pages or sections within the same page.
|
|
Title attribute
The title attribute specifies extra information about the element. The information is most often shown as a tooltip text when the mouse moves over the element.
|
|
Style attribute
The style attribute specifies an inline style for an element. It will override any style set globally, eg. styles specified in the <style>
tag or in an external style sheet.
The style attribute can also be used in any HTML element.
|
|
4. HTML Links🔗
Text links
Text links are created using <a>
anchor tag. The text within the anchor tags become clickable, directing users to the specified URL. To create a text link, use the following format:
|
|
Image links
We can also create link using images. To make an image clickable, place the <img>
tag within the <a>
tags. Here is the format:
|
|
Absolute URL
An absolute URL specifies the complete web address, including the protoclol (https://).
|
|
Relative URL
A Relative URL specifies the path to the file or location relative to the current webpage. example: “about.html” or “../contact.html”
|
|
Linking to a specific page section
To link to a specific section within the same webpage. you can use anchor links. Anchor links allow user to jump directly to a particular section by clicking on a link. They are created using the id attribute to identify the target with a preceding # symbol Example:
|
|
Linking to a specific page section on another page
|
|
Additional Link Attribute
- target : Specifies how the link opens. for example, you can set target="_blank" to open the link in the new tab or window.
- download : Specifies that the linked resourse should be downloaded when clicked. it is commonly used for file downloads.
|
|
5. HTML Lists📝
Unordered lists
Unordered lists <ul>
are used to present items without a specific order or sequence. Each item in the list is defined using <li>
(list item) tag.
|
|
- item 1
- item 2
- item 3
Ordered lists
Ordered lists<ol>
are used for items that have specific order or sequence. Like unordered lists, each item is defined using <li>
tag.
|
|
- Item 1
- Item 2
- Item 3
Nested lists
HTML allows you to nest lists within lists to create hierarchical structures. This is achieved by placing a complete list structure inside an individual list item <li>
. this technique is useful for creating subcategories or multi-level lists.
|
|
Nested Lists
- list item 1
- list item 1.1
- list item 1.2
- list item 1.3
- list item 2
- list item 3
Styling lists
HTML lists can be customized and styled using css styles, you can change the apperance of list markers, adjust spacing, and create unique visual representations.
|
|
6. HTML Tables🗓️
Basic table structure
Tables in HTML are created using the <table>
element. Inside the <table>
element, we have rows defined by the <tr>
(table row) element. Within each row we define table headers using the <th>
(table header) element or table cells using the <td>
(table data) element.
|
|
Basic Table Structure
Header 1 | Header 2 |
---|---|
Cell 1 | Cell 2 |
Cell 3 | Cell 4 |
Table headers
Table headers <th>
are used to define the headings of each column in a table. By default, table headers are bold and centered. They help provide context and improve the readability of the table.
|
|
Table headers
Name | Phone |
---|
Table cells
Table cells <td>
hold the actual data within a table. Each cell corresponds to a specific row and column intersection. Table cells provide the content for each column in the table.
|
|
Name | Phone | |
---|---|---|
sumit | sumit2011kmr@gmail.com | 6202757997 |
sumit | sumit2011kmr@gmail.com | 6202757997 |
Styling tables
You can customize the apperance of tables using css. by applying css styles to the <table>
, <th>
, and <td>
elements, you can change the table’s layout, font, color, spacing and more.
|
|
7. HTML Forms📝
Form structure
Forms are created using the <form>
element. Inside the <form>
element, we define form controls such as input fields, checkboxes, radio buttons, dropdowns, and buttons.
Example:
|
|
Methods for submitting form data: GET and POST
Labels
Label elements provide a descriptive text label for form controls, such as input fields, checkboxes, and radio buttons. Associating labels with form controls improves usability by providing visual and interactive cues.
|
|
Input fields
Input fields <input/>
allow users to enter data. They can be used for various types of user input, such as text, numbers, dates, emails, and more.
|
|
Variations of the textbox input field:
- text - Renders a simple textbox
- email - Renders a textbox for inputting emails
- password - Renders a textbox for inputting passwords
- number - Renders a textbox for inputting numbers
- date - Renders a textbox for inputting dates
- time - Renders a textbox for inputting time
Input fiels attributes
Attributes for all textbox variation elements:
- value - default value
- placeholder - placeholder text
- maxlength - maximum character length
- disabled - disables the input, values will not be submitted
- readonly - disables the input, values will be submitted
- size - visible width of an input element
- required - specifies a required field which the user must fill Attributes for number, date and time:
- min - Defines the minimum value
- max - Defines the maximum value The step attribute is specific to the number and time fields and specifies a stepping interval.
Checkbox and radio buttons
Checkboxes (<input type="checkbox" />
) and radio buttons (<input type="radio" />
) allow users to select multiple options (checkboxes) or choose a single option from a group (radio buttons). To specify default selection, you can use the checked attribute.
|
|
Select dropdowns
Select dropdowns <select>
provide users with a list of options from which they can choose. Supports multiple selection with the multiple attribute. To define default option, use selected attribute on <option>
element
|
|
Textarea
The <textarea>
element is used in HTML to create a multi-line text input field that the user can type into.
|
|
File upload
The <input type="file"/>
element renders a control for selecting and uploading files.
|
|
Specific attributes:
- accept - Specifies the types of files that the server accepts (image/png, image/jpeg).
- multiple - Enables the uploading of multiple files at once
|
|
Buttons
Buttons <button>
allow users to perform actions within the form, such as submitting the form or resetting input fields.
|
|
GET request
-
GET requests are primarily used to retrieve data from a server. When a client sends a GET request, it asks the server to provide a representation of a specific resource.
-
Data in a GET request is appended to the URL as query parameters.
-
GET requests are visible in the browser’s address bar, making them less secure for sensitive data transmission.
POST request
- POST requests are used to send data to the server to create or modify resources. When a client sends a POST request, it includes the data in the request body.
- POST requests are not visible in the browser’s address bar, providing better security for sensitive data transmission.
- Data in a POST request is included in the request body.
Difference and usecases
The main differences between POST and GET requests can be summarized as follows:
- GET requests are used for data retrieval, while POST requests are used for data submission or modification.
- GET requests append data to the URL as query parameters, while POST requests include data in the request body.
- GET requests are visible in the browser’s address bar, while POST requests are not.
Use GET requests when:
- Retrieving data from the server.
- Accessing public information that does not require sensitive data.
- Sending lightweight data or parameters.
Use POST requests when:
- Submitting form data or user input.
- Modifying data on the server.
- Sending sensitive data that should not be visible in the URL or browser history.
8. HTML Media Elements
Images
As already mentioned, images are displayed using the <img>
element. They enhance the visual appeal and convey information on your webpages.
Example:
|
|

Here are some commonly used image formats:
- JPEG: It’s best for photographs or images with lots of colors. JPEGs can be compressed considerably, which can result in a faster load time.
- PNG: It’s ideal for images that require transparency and higher quality. PNGs are usually larger than JPEGs and should be used sparingly.
- SVG: Ideal for vector graphics as they are resolution-independent and typically smaller in file size.
- WebP: A modern image format that provides superior lossless and lossy compression for images on the web.
Audio
Audio content can be embedded using the <audio>
element. It allows you to provide audio files playable directly on your webpages.
Example:
|
|
Video
Video content can be embedded using the <video>
element. It allows you to provide video files playable directly on your webpages.
Example:
|
|
Svg
SVGs, or Scalable Vector Graphics, are used to include vector-based images in your HTML document. They are resolution-independent and can scale without loss of quality, which makes them ideal for graphics like logos or icons. Example:
|
|
Iframes
An iframe is used to embed another HTML document within the current one. You can use this to include content from another website, like a YouTube video or a map from Google Maps. Example:
|
|
Enhancing accessibility
Provide meaningful alternative text (alt) for images to describe their content to users who rely on screen readers or when the image cannot be displayed. For video and audio content, include captions, transcripts, or audio descriptions to make them accessible to users with hearing impairments. Example:
|
|
9. HTML Semantics
Header
The <header>
element represents the introductory or navigational content at the top of a webpage or a specific section within a webpage.
|
|
Navigation
The <nav>
element represents a section of a webpage that contains navigation links allowing users to navigate through different areas or pages of a website.
|
|
Main
The <main>
element represents the main content of a webpage. It should contains unique content that is directly related to the purpose or central topic of the webpage.
|
|
Article
The <article>
element represents a self contained composition that can be independently distributed or reused. it can represent blog posts, news article, forum, posts and more.
|
|
Section
The <section>
element represents a standlone section within a document or a thematic grouping of content. It helps to organize and structure the content of a webpage.
|
|
Aside
The <aside>
element represents content that is related to the main content of a webpage. It can contain sidebars, pull quotes, advertisements or other supporting information.
|
|
Footer
THe <footer>
element represents the footer or the bottom section of a webpage. It typically contains the copywrite information, links to legal documents and contact details.
|
|
10. HTML Styles
CSS Syntax
CSS consists of rulesets that define how HTML elements should be styled. A ruleset consists of a selector and one or more property-value pairs. Examole:
|
|
Applying styles-Selectors
To apply styles to HTML elements you can use different type of selectors.
- Tag selector A tag selector slects elements based on their HTML tag name.
- Class selector A class selector selects elements based on the presence of a specific class attribute value.
- Id selector An Id selector selects a specific elements based on the unique ID attribute value.
|
|
Applying styles-Types
To apply styles to HTML you can use different CSS types
- Inline CSS Inline css is used to apply a unique style to a singl HTML element. It uses the style attribute of an HTML element. This method of insertion applies the CSS rules directly into the HTML file.
|
|
- Internal CSS
Internal css also known as embedded css. it is used to a whole HTML page. The CSS rules are put in a
<style>
block in the<head>
section of the HTML file.
Example:
|
|
- External CSS
External css involves creating a separate .css file and linking it to your HTML document. This method of insertion adds in minimizing code redundancy and promotes reusability.
Example:
- First create an external css file inside your project folder.(styles.css)
|
|
- Then, link this css file to your HTML document.
|
|
Cascading and specificity
CSS follows a cascading and specificity model. which means that multiple styles can be applied to an element, and the most specific style takes precedence.
Inline Styles : Inline styles are applied directly to individual HTML elements using the style attribute.
Example:
|
|
Specificity : The specificity of a selector determines its priority when conflicting styles are applied. inline styles have the highest specificity, followed by ID selectors, class selectors and tag selectors.
Common CSS properties
- CSS provides a wide range of properties to control the appearance of HTML elements.
- color : Specifies the text color.
- background-color : Sets the background color.
- font-family : Defines the font family to use for text.
- font-size : Specifies the font size.
- margin : Controls the outer spacing of an element.
- padding : Sets the inner spacing of an elemet.
- border : Defines the border style,width and color.
Example:
|
|
11. HTML Best Practices
Use proper indentation and formatting
Proper indentation and formatting make your HTML code more readable and easier to understand. Indent nested elements to clearly represent their hierarchy and use consistent spacing and line breaks.
Example:
|
|
Use semantic HTML elements
Utilize semantic HTML elements to provide meaning and structure to your content. Semantic elements like <header>, <nav>, <main>, <article>, <footer>
convey the purpose and role of each section, enhancing accessibility and search engine optimization.
Example:
|
|
Provide descriptive and accessible text alternatives
For images, videos, and other non-text content, always include descriptive alternative text (alt attribute) to provide information about the content for users who cannot see it. Use concise and meaningful descriptions that convey the purpose or context of the content.
Example:
|
|
Separate structure and presentation
Separate the structure (HTML) from the presentation (CSS) and behavior (JavaScript) of your webpages. Keep your HTML code focused on defining the content and structure, while styling and interactivity are handled through external CSS and JavaScript files.
Example:
|
|
Optimize performance
To improve website performance, minimize the use of unnecessary elements, attributes, and external resources. Use efficient techniques like compressing images, combining and minifying CSS and JavaScript files, and leveraging browser caching.
Example:
|
|
Validate your HTML
Regularly validate your HTML code using online validation tools or browser developer tools. Validating ensures that your code adheres to the HTML specifications and helps identify any errors or potential compatibility issues. Example:
|
|
Optimize images
The first step in optimizing images is choosing the correct file format.
- JPEG: It’s best for photographs or images with lots of colors. JPEGs can be compressed considerably, which can result in a faster load time.
- PNG: It’s ideal for images that require transparency and higher quality. PNGs are usually larger than JPEGs and should be used sparingly.
- SVG: Ideal for vector graphics as they are resolution-independent and typically smaller in file size.
- WebP: A modern image format that provides superior lossless and lossy compression for images on the web.
- Resize Images: Images should be resized to fit the layout of your website.
- Image Compression: Compression reduces the file size without noticeably degrading the quality of the image below an acceptable level.
- Use CSS Sprites: CSS sprites combine multiple images into one single image, which can significantly reduce HTTP requests and improve load times.
- Lazy Loading: Lazy loading is a technique that delays the loading of non-critical or non-visible content until the point of need.
- Use a CDN: Content Delivery Networks (CDNs) can cache your images across a network of servers worldwide, making your site faster for users around the globe.
12. ARIA Labels
Enhancing Accessibility in web development
Understanding ARIA Labels
ARIA labels are used to provide additional infomation to assistive technolory, such as screen readers, enabling a better user experiance for individuals with disabilities.
Example:
|
|
Understanding ARIA roles and properties
ARIA roles define the purpose or type of an element, while ARIA properties provide additional properties or characteristics to describe the element further.
Example:
|
|
Common use cases for ARIA Labels
- Describing complex or interactive elements:
|
|
- Enhancing link texts:
|
|
- Describing images and Non-text content:
|
|
Implementing ARIA Labels
- Use the aria-label attribute.
- Leverage ARIA roles and properties.
- Keep labels concise and descriptive.
- Test with assistive technologies.
13. SEO for html
HTML Meta tags
Meta tags in HTML are typically used within the <head>
section of an HTML document to define metadata about the page.
The following are key meta elements:
|
|
Example:
|
|
Microdata
Microdata is a specific type of syntax that can be added to HTML to create machine readable data from the contents of a webpage.
Microdata uses a set of new HTML arrribute to embed structured data within existing HTML content. The attribute are:
- itemscope : This attribute is a boolean attribute that declares a new item.
- itemtype : This attribute declares what kind of item is being defined. It works in conjunction with itemscope.
- itemprop : This attribute is used to add properties to an item.
- itemid : This attribute assign a unique identifier to an item, providing a globally unique ID foe the item being marked up.
Example:
|
|
SEO best practice
By implementing SEO best practice while writing HTML, You can improve the visibility of your web pages in search engine results.
- Use Semantic HTML Elements
- Incorporate ARIA Labels
- Optimize Meta Tags
- Optimize these meta tags by including relevant keywords
- Structure Content with Headings
- Optimize Image Alt Text