Single Linked List
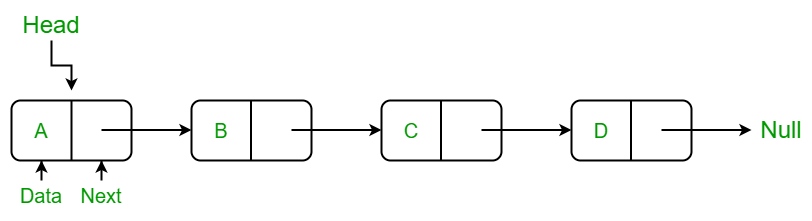
Navigation system works on the concept of linked list.
1. Introduction:
Single linked list is a linear data structure. It is slightly different from array. It made by the nodes
, each nodes consist a data and the address of the next node and each node
are linked using pointers. Here data are not stored in the contiguous memory location, nodes are present anywhere in the memory space but every nodes
are linked with eachother that’s why we call this linked list
. Main benefit of linked list is that we can insert or delete data from any position in the list.
2. Creation of Node
To create a new node in C++
, you will need to define a structure or class that represents a node, and then create an instance of that structure or class. Here we will use structure to create or define a node
.
Here is an example of a node structure in C++
or C
:
|
|
3. Insertion
An insertion operation in a single linked list involves adding a new node to the list. For this first you have to create a newnode
and then insert this node where you want. There are several ways to insert a node in the list:
- At the beginning of the list
- At the end of the list
- At a specific position of the list
3.1 Insertion at Begining
To insert a new node
at the beginning of the list, you need to create a newnode
and set it’s next pointer to point to the current head
of the list. Then, you can set the head
of the list to the newnode
. You also have to check whether the first node
is present or not in the list or we can say list is empty or not.
|
|
3.2 Insertion at Last
To insert a new node
at the end of the list, you need to traverse the list until you reach the last node
. Then, you can set the next pointer of the last node
to point to the newnode
. If the list is empty then set the head
to the newnode
.
temp
is just a temporarynode
just likecount
variable, after the operation it does’nt have any importance.
|
|
node
in a data structure, such as a linked list
, tree
, or graph
. There are several way to traverse. Here we use the simple traversal technique in which we form a temp
variable or node
and traverse the each node
one by one just pointing to the next pointer to the temp
.
temp=temp->next;
3.3 Insertion at Any Position
To insert a new node
at a desired position in the list, first you need to make a temporary node
say temp
initialise with head
then traverse the list until you reach the node
before the position where you want to insert the newnode
. Then, you can set the next pointer of the newnode
to point to the node
at the desired position, and set the next pointer of the node
before the position to point to the newnode
.
|
|
node
at that position.4. Deletion
A deletion operation in a single linked list involves removing a node
from the list and free the space. First make a temp
node, traverse to that node
and do the operation. There are several ways to delete a node
from the list:
- Deleting the head node
- Deleting the last node
- Deleting a node from the specific position of the list
4.1 Deletion from Begining
To delete the head
node or first node, first you have to make a temp
node initialise with head
then set the head
of the list to the next node
in the list. Now free the temp
.
|
|
4.2 Deletion from End
To delete the last node
, make a temp
node traverse the list until you reach the second-to-last node. Set the temp
to the last node
of the list. Then, you can set the next pointer of the second-to-last node
to null
. Now free the temp
.
|
|
4.3 Deletion from Any Position
To delete a node
in the middle of the list, you need to traverse the list until you reach the node
before the one you want to delete. Set the temp
to the node to be deleted in the list. Then, you can set the next pointer of the previous node
to point to the node
after the one you want to delete. Free the temp
.
|
|
node
from that position.5. Sorting
There are several way to sort the list.
|
|
6. Reversing
To reverse the linked list, You have to iterate the linked list and for each node
, changing the next pointer to point to the previous node
instead of the next node
as it traverses the linked list. This effectively reverses the order of the nodes in the linked list. The function returns the reversed linked list by returning the last node
in the original linked list as the new head
.
|
|
7. Print Function
To print the list you have to travarse the list and print each node
one by one. For this make a temp
node initialise with head
, travarse and print.
|
|
8. To Find Length
To find the length of the list, first make a temp
node initialise with head
than travarse the list and increment the count
by 1
on every travarsal until you reach to the last node. At last print the count
value.
|
|
9. Main Function
In the main function. we check all the function whether they are working or not, call all the functions one by one.
|
|
It will be a better practice if you take the input from the user in the output terminal. In this case the main function will be:
|
|
Complete Code of Single Linked List: Code
<div style="position: relative; padding-bottom: 56.25%; height: 0; overflow: hidden;">
<iframe allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture; web-share" allowfullscreen="allowfullscreen" loading="eager" referrerpolicy="strict-origin-when-cross-origin" src="https://www.youtube-nocookie.com/embed/FMzj9UYHTPQ?autoplay=0&controls=1&end=0&loop=0&mute=0&start=0" style="position: absolute; top: 0; left: 0; width: 100%; height: 100%; border:0;" title="YouTube video"
></iframe>
</div>